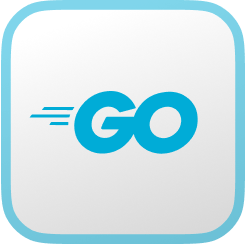
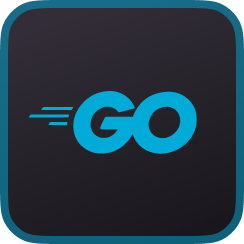
Golang SDK
Create Module
First we need to create the module if it does not exist yet:
go mod init github.com/$username/$projectname
Install the SDK
First, install the SurrealDB SDK using go get:
go get github.com/surrealdb/surrealdb.go
Connect to SurrealDB
Create a new main.go file and add the following code to try out some basic operations using the SurrealDB SDK.
package main
import (
"github.com/surrealdb/surrealdb.go"
)
type User struct {
ID string `json:"id,omitempty"`
Name string `json:"name"`
Surname string `json:"surname"`
}
func main() {
db, err := surrealdb.New("ws://localhost:8000/rpc")
if err != nil {
panic(err)
}
if _, err = db.Signin(map[string]interface{}{
"user": "root",
"pass": "root",
}); err != nil {
panic(err)
}
if _, err = db.Use("test", "test"); err != nil {
panic(err)
}
// Create user
user := User{
Name: "John",
Surname: "Doe",
}
// Insert user
data, err := db.Create("user", user)
if err != nil {
panic(err)
}
// Unmarshal data
createdUser := make([]User, 1)
err = surrealdb.Unmarshal(data, &createdUser)
if err != nil {
panic(err)
}
// Get user by ID
data, err = db.Select(createdUser[0].ID)
if err != nil {
panic(err)
}
// Unmarshal data
selectedUser := new(User)
err = surrealdb.Unmarshal(data, &selectedUser)
if err != nil {
panic(err)
}
// Change part/parts of user
changes := map[string]string{"name": "Jane"}
if _, err = db.Change(selectedUser.ID, changes); err != nil {
panic(err)
}
// Update user
if _, err = db.Update(selectedUser.ID, changes); err != nil {
panic(err)
}
if _, err = db.Query("SELECT * FROM $record", map[string]interface{}{
"record": createdUser[0].ID,
}); err != nil {
panic(err)
}
// Delete user by ID
if _, err = db.Delete(selectedUser.ID); err != nil {
panic(err)
}
}
SDK methods
The Golang SDK comes with a number of built-in functions.
Function | Description |
---|---|
surrealdb.New(url, options...) | Connects to a remote database endpoint. |
db.Close() | Closes the persistent connection to the database. |
db.Use(namespace, database) | Switch to a specific namespace and database. |
db.Signup(vars) | Switch to a specific namespace and database |
db.Signin(vars) | Signs in to a specific authentication scope. |
db.Invalidate() | Invalidates the authentication for the current connection |
db.Authenticate(token) | Authenticates the current connection with a JWT token |
db.Let(key, val) | Assigns a value as a parameter for this connection |
db.Query(sql, vars) | Runs a set of SurrealQL statements against the database |
db.Create(thing, data) | Creates a record in the database |
db.Select(what) | Selects all records in a table, or a specific record |
db.Update(what, data) | Updates all records in a table, or a specific record |
db.Change(what, data) | Modifies all records in a table, or a specific record |
db.Modify(what, data) | Applies JSON Patch changes to all records in a table, or a specific record |
db.Delete(what) | Deletes all records, or a specific record |
surrealdb.Unmarshal(data, v) | Unmarshal loads a SurrealDB response into a struct. It is perfectly fine to use this function however it requires casting types. |
surrealdb.UnmarshalRaw(rawData, v) | UnmarshalRaw loads a raw SurrealQL response returned by Query into a struct. This is not the same as a query result. The value of this function will include additional information such as execution time and status - details that tend to be omitted from the SurrealQL query the user would be interested in. |
.New()
Connects to a remote database endpoint.
Method Syntaxsurrealdb.New(url, options...)()
Arguments
Arguments | Description | ||
---|---|---|---|
url | The URL of the database endpoint to connect to. Examples may include http://hostname:8000 or ws://hostname:8000/rpc . | ||
options | Set SurrealDB clients options such as Timeout etc. |
Example usage
// Connect to a local endpoint
surrealdb.New("ws://localhost:8000/rpc");
// Connect to a remote endpoint
surrealdb.New("ws://cloud.surrealdb.com/rpc");
.Close()
Closes the persistent connection to the database.
Method Syntaxdb.Close()
Example usage
db.Close();
.Use()
Switch to a specific namespace and database.
Method Syntaxdb.Use(namespace, database)
Arguments
Arguments | Description | ||
---|---|---|---|
ns | Switches to a specific namespace. | ||
db | Switches to a specific database. |
Example usage
db.Use("happy-hippo-staging", "my-service")
.Signup()
Signs up to a specific authentication scope.
Method Syntaxdb.Signup(vars)
Arguments
Arguments | Description | ||
---|---|---|---|
vars | Variables used in a signup query. |
Example usage
db.Signup(map[string]string{
"NS": "clear-crocodile-production",
"DB": "web-scraping-application",
"SC": "user",
"email": "info@surrealdb.com",
"pass": "123456",
})
.Signin()
Signs in to a specific authentication scope.
Method Syntaxdb.Signin(vars)
Arguments
Arguments | Description | ||
---|---|---|---|
vars | Variables used in a signin query. |
Example usage
db.Signin(map[string]string{
"user": "root",
"pass": "root",
})
.Invalidate()
Invalidates the authentication for the current connection. Invalidates close the authenticated connection.
Method Syntaxdb.Invalidate()
Example usage
db.invalidate();
.Authenticate()
Authenticates the current connection with a JWT token.
Method Syntaxdb.Authenticate(token)
Arguments
Arguments | Description | ||
---|---|---|---|
token | The JWT authentication token. |
Example usage
db.Authenticate("eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJTdXJyZWFsREIiLCJpYXQiOjE1MTYyMzkwMjIsIm5iZiI6MTUxNjIzOTAyMiwiZXhwIjoxODM2NDM5MDIyLCJOUyI6InRlc3QiLCJEQiI6InRlc3QiLCJTQyI6InVzZXIiLCJJRCI6InVzZXI6dG9iaWUifQ.N22Gp9ze0rdR06McGj1G-h2vu6a6n9IVqUbMFJlOxxA");
.Let()
Assigns a value as a parameter for this connection.
Method Syntaxdb.Let(key, val)
Arguments
Arguments | Description | ||
---|---|---|---|
key | Specifies the name of the variable. | ||
val | Assigns the value to the variable name. |
Example usage
// Assign the variable on the connection
db.Let("name", map[string]string{
"first": "ElecTwix",
"last": "Morgan Hitchcock",
});
// Use the variable in a subsequent query
db.Query("CREATE person SET name = $name", nil);
// Use the variable in a subsequent query
db.Query("SELECT * FROM person WHERE name.first = $name.first", nil);
.Query()
Runs a set of SurrealQL statements against the database.
Method Syntaxdb.Query(sql, vars)
Arguments
Arguments | Description | ||
---|---|---|---|
sql | Specifies the SurrealQL statements. | ||
vars | Assigns variables which can be used in the query. |
Example usage
// Assign the variable on the connection
result, err := db.Query("CREATE person; SELECT * FROM type::table($tb);", map[string]string{
"tb": "person"
});
.Create()
Creates a record in the database.
Method Syntaxdb.Create(thing, data)
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or the specific record ID to create. | ||
data | The document / record data to insert. |
Example usage
// Create a record with a random ID
db.Create("person", map[string]interface{}{})
// Create a record with a specific ID w/ a map
db.Create("person:tobie", map[string]interface{}{
"name": "Tobie",
"settings": map[string]bool{
"active": true,
"marketing": true,
},
})
// Create a record with a specific ID w/ a struct
type Person struct {
Name string
Surname string
Settings settings
}
type settings struct {
Active bool
Marketing bool
}
data := Person{
Name: "Hugh",
Settings: settings{
Active: true,
Marketing: true,
},
}
db.Create("person:hugh", data)
Translated query
This function will run the following query in the database:
CREATE $thing CONTENT $data;
.Select()
Selects all records in a table, or a specific record, from the database.
Method Syntaxdb.Select(what)
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or a record ID to select. |
Example usage
// Select all records from a table
db.Select("person");
// Select a specific record from a table
db.Select("person:h5wxrf2ewk8xjxosxtyc");
Translated query
This function will run the following query in the database:
SELECT * FROM $thing;
.Update()
Updates all records in a table, or a specific record, in the database.
Method Syntaxdb.Update(what, data)
NOTE: This function replaces the current document / record data with the specified data.
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or the specific record ID to update. | ||
data | The document / record data to insert. |
Example usage
// Update all records in a table
db.Update("person");
// Update a record with a specific ID
db.Update("person:ElecTwix", map[string]interface{}{
"name": "ElecTwix",
"settings": map[string]bool{
"active": true,
"marketing": true,
},
});
Translated query
This function will run the following query in the database:
UPDATE $thing CONTENT $data;
.Change()
Modifies all records in a table, or a specific record, in the database.
Method Syntaxdb.Change(what, data)
NOTE: This function merges the current document / record data with the specified data.
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or the specific record ID to change. | ||
data | The document / record data to insert. |
Example usage
// Update all records in a table
db.Change("person", map[string]interface{}{
"updated_at": time.Now(),
});
// Update a record with a specific ID
db.Change("person:tobie", map[string]interface{}{
"updated_at": time.Now(),
"settings": map[string]bool{
"active": true,
},
});
Translated query
This function will run the following query in the database:
UPDATE $thing MERGE $data;
.Modify()
Applies JSON Patch changes to all records, or a specific record, in the database.
Method Syntaxdb.Modify(what, data)
NOTE: This function patches the current document / record data with the specified JSON Patch data.
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or the specific record ID to modify. | ||
data | The JSON Patch data with which to modify the records. |
Example usage
// Update all records in a table
db.Modify("person", []surrealdb.Patch{op: "replace", path: "/created_at", value: time.Now()})
// Update a record with a specific ID
db.Modify("person:tobie", []surrealdb.Patch{
{op: "replace", path: "/settings/active", value: false},
{op: "add", path: "/tags", value: []string{"developer", "engineer"}},
{op: "remove", path: "/temp"},
})
Translated query
This function will run the following query in the database:
UPDATE $thing PATCH $data;
.Delete()
Deletes all records in a table, or a specific record, from the database.
Method Syntaxdb.Delete(resource)
Arguments
Arguments | Description | ||
---|---|---|---|
thing | The table name or a record ID to select. |
Example usage
// Delete all records from a table
db.Delete("person");
// Delete a specific record from a table
db.delete("person:h5wxrf2ewk8xjxosxtyc");
Translated query
This function will run the following query in the database:
DELETE * FROM $thing;
.Unmarshal()
Unmarshal loads a SurrealDB response into a struct. This function does not use generics.
Method Syntaxsurrealdb.Unmarshal(data, target)
Arguments
Arguments | Description | ||
---|---|---|---|
data | The response from other queries. | ||
target | The target struct to unmarshal the data into. |
Example usage
// a simple user struct
type testUser struct {
surrealdb.Basemodel `table:"test"`
Username string `json:"username,omitempty"`
Password string `json:"password,omitempty"`
ID string `json:"id,omitempty"`
}
// select a user
userData, err := db.Select("person:electwix")
var user testUser
err = surrealdb.Unmarshal(userData, &user)
.UnmarshalRaw()
UnmarshalRaw loads a raw SurrealQL response returned by Query into a struct. The raw response includes metadata that was not part of the intended query, such as duration of query.
Method Syntaxsurrealdb.UnmarshalRaw(rawData, target)
Arguments
Arguments | Description | ||
---|---|---|---|
rawData | Raw Query response. | ||
target | The target struct that will be unmarshalled to. |
Example usage
// a simple user struct
type testUser struct {
Username string `json:"username,omitempty"`
Password string `json:"password,omitempty"`
ID string `json:"id,omitempty"`
}
username := "johnny"
password := "123"
// create test user with raw SurrealQL and unmarshal
userData, err := db.Query("create users:johnny set Username = $user, Password = $pass", map[string]interface{}{
"user": username,
"pass": password,
})
var userSlice []testUser
ok, err := surrealdb.UnmarshalRaw(userData, &userSlice)