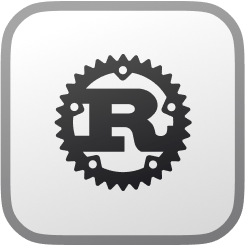
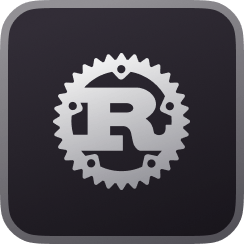
Rust SDK
Install the SDK
First, create a new project using cargo new and add the SurrealDB SDK to your dependencies:
cargo add surrealdb
Connect to SurrealDB
Open src/main.rs and replace everything in there with the following code to try out some basic operations using the SurrealDB SDK.
use serde::{Deserialize, Serialize};
use surrealdb::engine::remote::ws::Ws;
use surrealdb::opt::auth::Root;
use surrealdb::sql::Thing;
use surrealdb::Surreal;
#[derive(Debug, Serialize)]
struct Name<'a> {
first: &'a str,
last: &'a str,
}
#[derive(Debug, Serialize)]
struct Person<'a> {
title: &'a str,
name: Name<'a>,
marketing: bool,
}
#[derive(Debug, Serialize)]
struct Responsibility {
marketing: bool,
}
#[derive(Debug, Deserialize)]
struct Record {
#[allow(dead_code)]
id: Thing,
}
#[tokio::main]
async fn main() -> surrealdb::Result<()> {
// Connect to the server
let db = Surreal::new::<Ws>("127.0.0.1:8000").await?;
// Signin as a namespace, database, or root user
db.signin(Root {
username: "root",
password: "root",
})
.await?;
// Select a specific namespace / database
db.use_ns("test").use_db("test").await?;
// Create a new person with a random id
let created: Vec<Record> = db
.create("person")
.content(Person {
title: "Founder & CEO",
name: Name {
first: "Tobie",
last: "Morgan Hitchcock",
},
marketing: true,
})
.await?;
dbg!(created);
// Update a person record with a specific id
let updated: Option<Record> = db
.update(("person", "jaime"))
.merge(Responsibility { marketing: true })
.await?;
dbg!(updated);
// Select all people records
let people: Vec<Record> = db.select("person").await?;
dbg!(people);
// Perform a custom advanced query
let groups = db
.query("SELECT marketing, count() FROM type::table($table) GROUP BY marketing")
.bind(("table", "person"))
.await?;
dbg!(groups);
Ok(())
}
To run the example above, you will need to add the following additional dependencies:
cargo add serde --features derive
cargo add tokio --features macros,rt-multi-thread
Then make sure your SurrealDB server is running on 127.0.0.1:8000 and run your app from the command line with:
cargo run
SDK methods
The Rust SDK comes with a number of built-in functions.
Function | Description |
---|---|
Surreal::init() | Initialises a static database engine |
db.connect(endpoint) | Connects to a specific database endpoint, saving the connection on the static client |
Surreal::new::<T>(endpoint) | Connects to a local or remote database endpoint |
db.use_ns(namespace).use_db(database) | Switch to a specific namespace and database |
db.signup(credentials) | Signs up a user to a specific authentication scope |
db.signin(credentials) | Signs this connection in to a specific authentication scope |
db.invalidate() | Invalidates the authentication for the current connection |
db.authenticate(token) | Authenticates the current connection with a JWT token |
db.set(key, val) | Assigns a value as a parameter for this connection |
db.query(sql) | Runs a set of SurrealQL statements against the database |
db.select(resource) | Selects all records in a table, or a specific record |
db.select(resource).live() | Initiate live queries for live stream of notifications |
db.create(resource).content(data) | Creates a record in the database |
db.update(resource).content(data) | Updates all records in a table, or a specific record |
db.update(resource).merge(data) | Modifies all records in a table, or a specific record |
db.update(resource).patch(data) | Applies JSON Patch changes to all records in a table, or a specific record |
db.delete(resource) | Deletes all records, or a specific record |
.init()
The DB static singleton ensures that a single database instance is available across very large or complicated applications. With the singleton, only one connection to the database is instantiated, and the database connection does not have to be shared across components or controllers.
Method SyntaxSurreal::init()
Example usage
static DB: Lazy<Surreal<Client>> = Lazy::new(Surreal::init);
#[tokio::main]
async fn main() -> surrealdb::Result<()> {
// Connect to the database
DB.connect::<Wss>("cloud.surrealdb.com").await?;
// Select a namespace + database
DB.use_ns("test").use_db("test").await?;
// Create or update a specific record
let tobie: Option<Record> = DB
.update(("person", "tobie"))
.content(Person { name: "Tobie" })
.await?;
Ok(())
}
.connect()
Connects to a local or remote database endpoint.
Method Syntaxdb.connect(endpoint)
Arguments
Arguments | Description | ||
---|---|---|---|
endpoint | The database endpoint to connect to. |
Example usage
// Connect to a local endpoint
DB.connect::<Ws>("127.0.0.1:8000").await?;
// Connect to a remote endpoint
DB.connect::<Wss>("cloud.surrealdb.com").await?;
.new()
Connects to a local or remote database endpoint.
Method SyntaxSurreal::new::<T>(endpoint)
Arguments
Arguments | Description | ||
---|---|---|---|
endpoint | The database endpoint to connect to. |